Challenge: Dynamically determining shipping costs based on cart contents and destination
Solution: Retrieving shipping costs using the calculate_shipping method
Welcome to another insightful installment of our “WooCode Tips” series, where we delve into the mysteries of WooCommerce, offering programmers like you a treasure trove of hacks and tricks.
Today, we’re tackling a common yet complex challenge faced by many WooCommerce store owners: calculating shipping costs programmatically based on the specific contents of a customer’s cart and their destination.
Background
Imagine a scenario where your WooCommerce store needs to dynamically adjust shipping costs. This isn’t just about applying a flat rate or a simple rule; it’s about understanding and responding to the unique combination of items in a customer’s basket and where those items are headed.
In this post, we’re going to create a custom PHP function designed to fetch real-time shipping costs based on the current state of the customer’s cart and their shipping destination.
Typically, WooCommerce stores rely on predefined shipping methods and rates, which can range from simple flat rates to more complex table rate shipping based on weight, size, or destination. However, these standard methods often fall short when dealing with dynamic and diverse cart contents or specific customer needs.
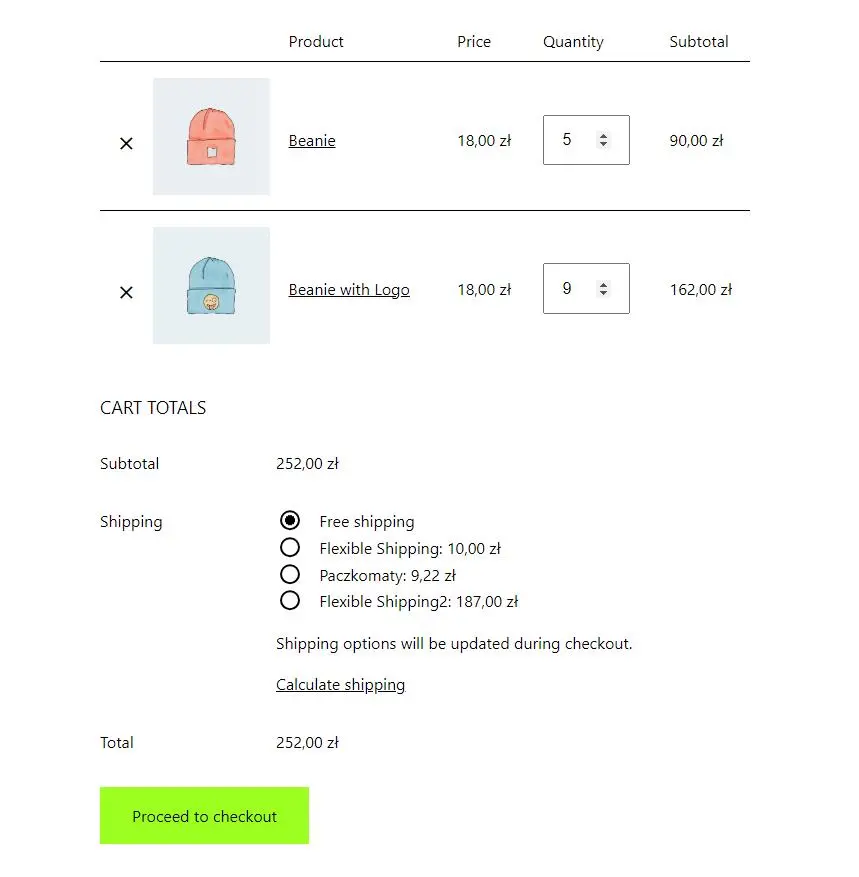
Fetching shipping costs
Our solution is centered around a custom PHP function, ct_get_available_shipping_methods_for_customer_cart. It takes the unique contents of a customer’s cart and their shipping destination to calculate shipping costs accurately and dynamically. Here’s how it works:
Fetching cart contents: The function starts by checking if WooCommerce is active and whether the cart contains items. If the cart is empty, there’s no need to calculate shipping, so the function returns an empty array.
function ct_get_available_shipping_methods_for_customer_cart($country_code = 'PL') { if (function_exists('WC')) { if (!WC()->cart || WC()->cart->is_empty()) { return array(); } $enabled_methods = array();
Creating a package from the cart: the function converts the cart contents into a ‘package’, a data structure that WooCommerce understands and can use for shipping calculations. This package includes details of the items in the cart and their destination, which in this case, is dynamically set based on the $country_code parameter.
$package = WC()->cart->get_shipping_packages()[0]; // Assuming single package $package['destination'] = array('country' => $country_code);
Retrieving shipping methods for the destination zone: WooCommerce categorizes shipping destinations into zones. Our function iterates through these zones to find the one matching the destination country code. Once the matching zone is found, the function retrieves all available shipping methods for that zone.
$zones = WC_Shipping_Zones::get_zones(); foreach ($zones as $zone) { $matching_locations = array_filter($zone['zone_locations'], function ($location) use ($country_code) { return $location->code == $country_code; }); if (!empty($matching_locations)) { $shipping_zone = new WC_Shipping_Zone($zone['id']); $available_methods = $shipping_zone->get_shipping_methods(true); foreach ( $available_methods as $method ) { if ( $method->is_enabled() ) { // fetch costs } } } }
Returning shipping methods and costs: The function concludes by returning an array of enabled shipping methods along with their calculated costs.
else { $method_id = get_class($method); $enabled_methods[] = array( 'method_title' => $method->get_method_title(), 'method_id' => $method_id, 'cost' => floatval($method->get_option('cost')), ); } return $enabled_methods;
Testing the function
To ensure our custom function works as intended, it’s crucial to test it thoroughly. Here’s how you can test the ct_get_available_shipping_methods_for_customer_cart function:
Triggering the function via a browser
We’ll use a small script to trigger our function through a browser request. Add the following PHP code snippet to your WooCommerce site:
add_action('wp_loaded', 'ct_test_script_1'); function ct_test_script_1() { $param_name = 'ct_trigger_shipping_methods_info'; if (isset($_GET[$param_name]) && !empty($_GET[$param_name])) { $shipping_methods = ct_get_available_shipping_methods_for_customer_cart(); header('Content-Type: application/json'); echo json_encode($shipping_methods); exit; // Stop further execution to prevent loading the full page } }
This code sets up a WordPress action that triggers when the page loads. It checks for a specific GET parameter in the URL (/?ct_trigger_shipping_methods_info=123) and, if present, executes our shipping method function, returning the results in JSON format.
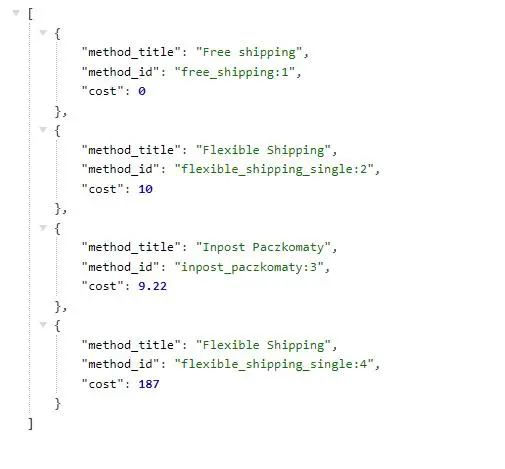
Integration with the Flexible-shipping plugin
Now, let’s discuss how our custom function integrates with the popular ‘Flexible-shipping’ plugin for WooCommerce. This plugin is renowned for its ability to calculate shipping costs based on various factors like weight, cart total, and more.
The Flexible-shipping plugin, developed by Octolize, has been used by over 100,000 WooCommerce stores. Its free version offers robust features, while the PRO version takes shipping customization to another level with support for shipping classes, conditional logic, additional costs, and more.
Our function seamlessly interacts with the Flexible-shipping plugin. It uses the calculate_shipping method from the plugin to determine the shipping cost based on the rules set by the store admin. This integration ensures that the shipping costs are dynamically calculated using the latest rules and conditions defined in the plugin.
Calculating flexible-shipping costs programmatically
The calculate_shipping method of the Flexible-shipping plugin plays a crucial role here. It takes the prepared package as input and applies the shipping rules defined in the WooCommerce settings. These rules can include factors like weight, dimensions, and cart total, offering a highly customizable approach to shipping cost calculation.
Once the calculate_shipping method is called, it returns the shipping rates based on the package data and the applicable rules. These rates are then stored in an array, providing a detailed breakdown of the shipping options and costs.
Code snippet example
// Assuming a single package $package = WC()->cart->get_shipping_packages()[0]; $package['destination'] = array('country' => $country_code); //... (other code) if (method_exists($method, 'calculate_shipping')) { $method->calculate_shipping($package); $rates = $method->rates; foreach ($rates as $rate) { $enabled_methods[] = array( 'method_title' => $method->get_method_title(), 'method_id' => $rate->get_id(), 'cost' => floatval($rate->get_cost()), ); } }
Example script output
[ { "method_title": "Free shipping", "method_id": "free_shipping:1", "cost": 0 }, { "method_title": "Flexible Shipping", "method_id": "flexible_shipping_single:2", "cost": 10 }, { "method_title": "Flexible Shipping", "method_id": "flexible_shipping_single:4", "cost": 187 } ]
Advanced features and PRO version of Flexible-shipping
PRO version of the Flexible-shipping plugin opens up a world of advanced possibilities. Let’s explore some of these features and how they can further enhance your WooCommerce store’s shipping capabilities:
Advanced shipping options
The PRO version supports a wide range of advanced features, including shipping costs based on product dimensions, volume, dimensional weight, and more. These features allow for even more precise shipping cost calculations, catering to a variety of products and shipping scenarios.
Conditional logic and additional costs
With conditional logic, you can create complex shipping rules that match your specific business needs. For instance, you can adjust shipping costs based on user roles, time of day, or day of the week. Additional costs can be applied based on various parameters like price, weight, and item quantity, offering further customization.
Stopping and hiding shipping methods
A unique feature of the PRO version is the ability to stop a rule from being calculated or hide a shipping method based on certain conditions. This allows for greater control over the shipping options presented to the customer.
Free shipping coupons support
The PRO version also supports free shipping coupons, adding another layer of customer engagement and marketing potential to your store.
Additional resources
For those seeking to deepen their knowledge and expertise in WooCommerce shipping calculations and related topics, the following resources will prove invaluable:
- WooCommerce documentation: The official WooCommerce documentation about setting up and managing shipping options. It’s a must-visit for anyone working with WooCommerce. WooCommerce Shipping Documentation.
- Flexible Shipping plugin – Free Vs PRO Comparison: Understand the differences and added advantages of the PRO version over the free version of the Flexible-shipping plugin. This comparison will help you make an informed decision on which version suits your needs. Flexible Shipping – Free Vs PRO – Features Comparison.
- GitHub Gist: For a direct look at the custom PHP function we discussed, visit our GitHub Gist. This Gist provides the complete code that you can use or modify for your WooCommerce store. Access it here.
Looking for a talented team to bring your dream project to life? Contact us.
Comments
0 response