Implementing a character counter in React
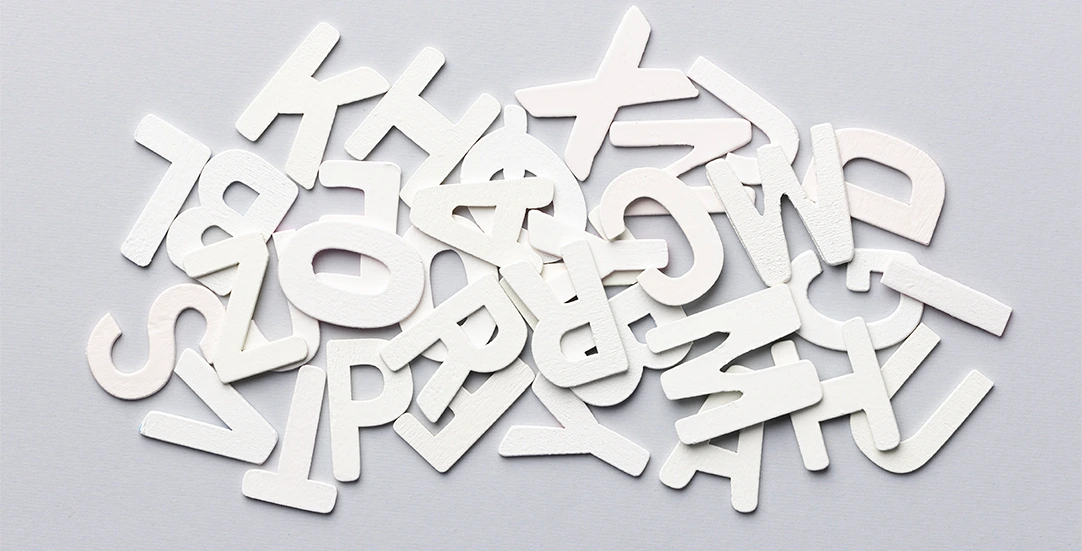
CHALLENGE: create a React character counter with a character limit for text area and display current count
SOLUTION: handle the onChange event, use a Bootstrap badge to display the results
Sometimes we want to restrict the number of characters that can be written in text input or text area. One solution is to use the HTML5 attribute for form elements, like maxlength. For example:
<Form.Control as="textarea" maxLength={characterLimit} />
However, it will be even better to display the current character count and when the limit is exceeded to change the color of a form element to red. We will be using the isInvalid parameter of Form.Control and changing the badge background to danger to emphasize that the limit has been achieved.
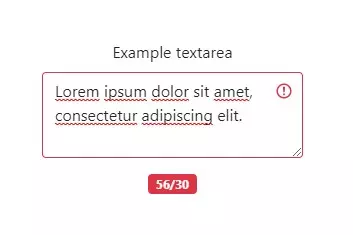
React Bootstrap
To have general styles and invalid states already styled, we’re going to install the NPM library with Bootstrap components. Use the following command to install dependency:
npm install react-bootstrap bootstrap@5.1.3
And then, import styles in src/index.jsx :
// src/index.jsx
import 'bootstrap/dist/css/bootstrap.min.css';
We use basic Grid elements, Form for displaying textarea and badge for the character counter display.
Demo of Character Counter React
Here is the source code for the main application. When the user is typing in the textarea, we’re updating the inputText value. Having this, we can easily calculate the length (number of characters typed). CharacterLimit is set to 30. An error will appear after exceeding the value.
// src/App.jsx
import React, { useState } from "react";
import './App.css';
import Container from 'react-bootstrap/Container'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Form from 'react-bootstrap/Form'
import Badge from 'react-bootstrap/Badge'
function App() {
const [inputText, setInputText] = useState("");
const [characterLimit] = useState(30);
// event handler
const handleChange = event => {
setInputText(event.target.value);
};
return (
<div className="App">
<Container>
<Row className="justify-content-md-center">
<Col xs lg="3">
<Form>
<Form.Group className="mb-3" controlId="exampleForm.ControlTextarea1">
<Form.Label>Example textarea</Form.Label>
<Form.Control as="textarea" rows={3} value={inputText} onChange={handleChange} isInvalid={(inputText.length > characterLimit)} />
<Badge className='mt-3' bg={`${inputText.length > characterLimit ? 'danger' : 'primary'}`}>{inputText.length}/{characterLimit}</Badge>
</Form.Group>
</Form>
</Col>
</Row>
</Container>
</div>
);
}
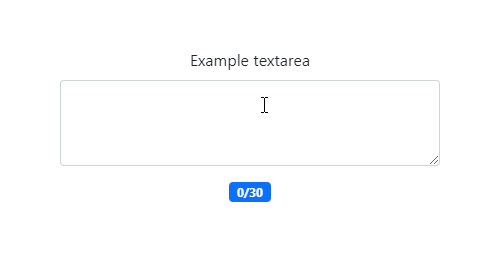
export default App;
That’s it for today’s tutorial, make sure to follow us for other useful tips and guidelines, and don’t forget to subscribe to our newsletter.
Do you need someone to implement this react character counter on any other solution for you? Check out our specialists for hire in the outsourcing section. You can also check our custom web development page if you need single service from professional team of developers!