Symfony – testing emails in the browser
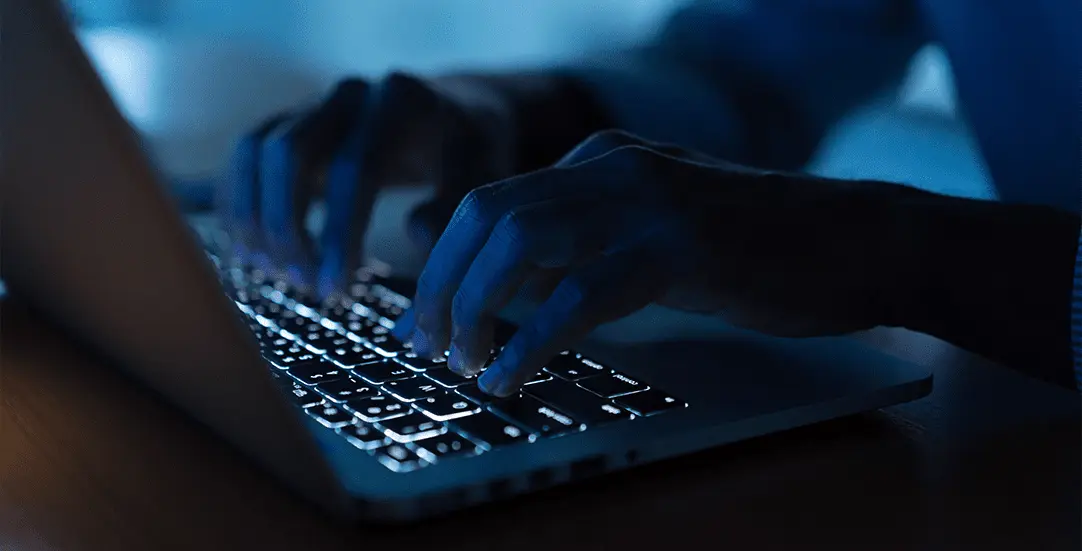
Symfony 4.4, popular PHP framework, can be configured to use Swift Mailer library. Configuration can be defined in swiftmailer.yaml file: sending using own SMTP server or other email providers like: SendGrid, Amazon SES or Gmail. For Advanced User Management – FOSUserBundle can be used. It provides user registration and password recovery, including “confirmation per email” functionality.
Testing emails
Testing email templates rendering can be tricky. Often system notifications are triggered on special conditions / time intervals. In those situations tests can be time-consuming or not thorough enough.
Sending to a Specified Email Address
You can force symfony to send all notifications to your e-mail.
# config/packages/dev/swiftmailer.yaml swiftmailer: delivery_addresses: ['dev@example.com']
Email Testing Tools
Other solution for testing emails are SMTP applications that can catch any message sent and display it in web interface. Most popular open-source solutions for SMTP testing are: MailCatcher (https://github.com/sj26/mailcatcher) and MailHog (https://github.com/mailhog/MailHog)
Emails in Symfony
Symfony 4 provides functionality to define email templates using TWIG templates. Common parts as header, footer are defined as blocks. Sometimes you want to display image in the email, you can embed them or link using url. Embedding images will make email bigger and can negatively affect deliverability. We are going to use SendGrid Image Editor, which is adding images to CDN. Image uploaded to CDN – we can use in symfony TWIG templates.
Automated email testing
It is recommended to test email rendering in the most popular Clients like: Apple iPhone, Gmail, Apple Mail, Outlook, Yahoo! Mail and Google Android. Previewing email design, SPAM score verification, checking sender reputation – are the functionalities provided by online services like Litmus, Mailtrap, or Email on Acid. By optimizing the emails you will increase email open rate and minimize possibility to mark it as SPAM.
Preview emails in the browser
Imagine – that you can use one url: /emails-preview – to immediately see all emails from the Symfony PHP system. Every change done in html.twig email templates will be visible on the preview link. We’re going to loop through all the templates, pass needed twig variables and render HTML source.
<?php /** * * @Route("/emails-preview", name="my_app_emails_preview", options={"expose"=true}) * @param Request $request * @return JsonResponse */ public function emailPreview(Request $request): Response { $env = $this->getParameter('kernel.environment'); if($env != 'dev') { return new Response("Forbidden", 403); } // register confirmation $user = $this->getUser(); $tokenTest = 'FZlLTNfly1C0RnrB1lNSPIt8G1gj46eTfdBaTFNE'; $user->setConfirmationToken($tokenTest); // results $userResults = array( array("event" => array("name" => "Event test 1234"), "users" =>"User1, User2, User3"), array("event" => array("name" => "Some Event lorem ipsum"), "users" =>"User 4"), ); // prices $pricePack = [ 'value' => 4.99, 'currency' => ['code'=> 'USD'] ]; $templates = [ // TESTING 'home/_emails/dev/testing.html.twig', // FOS USER BUNDLE 'app/_emails/registration/confirmation.html.twig', 'app/_emails/account/delete_account.html.twig', 'app/_emails/password/recovery.html.twig', 'bundles/FOSUserBundle/Resetting/password_resetting.html.twig', 'app/_emails/contact/contact.html.twig', 'app/_emails/career/job.html.twig', // EVENTS 'app/_emails/event/event1.twig', 'app/_emails/event/event2.twig', 'app/_emails/event/event3.twig', 'app/_emails/event/event4.twig', 'app/_emails/event/event5.twig', 'app/_emails/event/event6.twig', 'app/_emails/event/event7.twig', 'app/_emails/event/event8.twig', 'app/_emails/event/event9.twig', 'app/_emails/event/event10.twig', 'app/_emails/event/event11.twig', 'app/_emails/event/event12.twig', 'app/_emails/event/event13.twig', 'app/_emails/event/event14.twig', 'app/_emails/event/event15.twig', // USER 'app/_emails/user/notification1.html.twig', 'app/_emails/user/notification2.html.twig', 'app/_emails/user/notification3.html.twig', 'app/_emails/user/notification4.html.twig', 'app/_emails/user/notification5.html.twig', 'app/_emails/user/notification6.html.twig', 'app/_emails/user/notification7.html.twig', ]; // set language $request->getSession()->set('_locale', 'EN_en'); $result = ''; $counter = 0; foreach($templates as $key => $item): $counter++; if($counter != 1) { // continue; } $result .= '<h2 style="color:#fff; font-size:18px; padding:20px 3px 3px 3px; display:flex; justify-content: center; align-items: center; border-top:2px solid #333; "> <span style="height:30px; width:30px; line-height: 30px; font-weight: bold; border-radius:50%; background:#333; color:#fff; margin-right:5px;font-size: 12px; text-align: center;">'.$counter . '</span>'. $item . '</h2>'; $result .= $this->renderView( $item, [ 'link' => 'https://www.example.com', 'description' => 'some description 123', 'job' => 'jobName 56', 'salaryExpectation' => '23', 'phone' => '323 44 5 666', 'email_address' => 'dsadada@example.com', 'lastName' => 'Lorem ips 34', 'firstName' => 'My name 123', 'pricePack' => $pricePack, 'friend' => $user, 'userResults' => $userResults, 'sender' => $user, 'receiver' => $user, 'message' => 'Some message here lorem ipsum', 'subject' => "Some subject lorem ipsum", 'confirmationUrl' => 'https://www.example.com/resetting/reset/-T-FZlLTNfly1C0RnrB1lNSPIt8G1gj46eTfdBaTFNE', 'token' => $tokenTest, 'username' => $user, 'user' => $user, ] ); endforeach; return new Response( $result ); }
About us:
Hi, we are createIT!
As a passionate group of professionals with a love for Web and mobile technologies, we have been successfully serving our clients for the past 15 years. Through these years we have continuously striven to create the best IT solutions our clients seek.
Our 70+ developer team creates cutting-edge complex B2B and B2C web systems and apps as well as delivers top-notch direct outsourcing services.
We work in multiple frameworks but we hold special feelings and are experts in PHP (Symfony, OXID, WordPress), JavaScript (React, AngularJS), Flutter and Blockchain. We do enjoy working in the scalable cloud environment like AWS.
We are located in Warsaw, Poland, in the heart of Europe. Concentrated on delivering durable web solutions of high integrity, we do not always try to be the cheapest. However, thanks to our location you will be surprised how reasonable our prices are.
Fluent in English we offer services worldwide. Among others, in countries such as the U.S.A., Great Britain, Germany, Australia, Sweden and Poland.